How I Used Design Patterns in Arcane Lands (Part I)
- Justin San Juan
- Oct 27, 2019
- 2 min read
In the development of this video-game, I have applied the following patterns:
Factory Pattern
Strategy Pattern
Observer Pattern
Composite
These Design Patterns are methods used to enhance the flexibility and maintainability of the program. This is mostly achieved by delegating the work done on concepts that vary to new objects, which I will explain further later on.
Another concept to note is creating a framework vs a library in the game. A library is a set of tools with specific functions that a developer uses, meanwhile a framework is like a set of events that are triggered on behalf of the framework, and the developer decides what occurs in these events. An example of framework events are OnChange in the usual JavaScript web pages.
In this game, certain events such as OnHit for a character would need to be implemented in different ways for different characters.
Factory Pattern
In the game, I used the factory pattern to delegate the work of creating a new enemy instance to a static class in C#. In particular, I use a parameterized factory pattern to generate different kinds of enemies, where the id is a enumeration. In the image below, the factory will return an object that implements the IEnemy interface. Each type of enemy (Knight, Wizard, Archer) moves in various ways and fires in different patterns, thus they would implement Fire() and Move() differently.
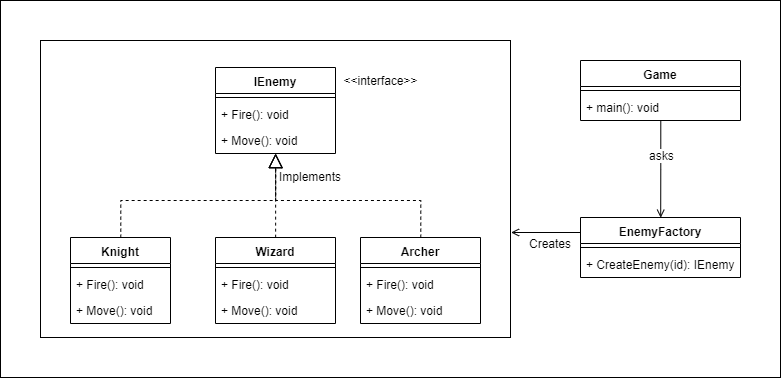
Comparing this with the naive method of hard-coding enemy generation, without the IEnemy interface, one might have to write:
Archer archer = new Archer();
archer.Move();
Meanwhile, using the factory pattern with a base interface (or abstract class) defined, one would then write:
IEnemy enemy = EnemyFactory.GetEnemy(id);
enemy.Move();
(where id is EnemyType.Archer)
The important point to note is that Archer does not appear in the actual enemy creation. This makes the code more flexible as we then program to an interface (IEnemy), rather than an implementation (Archer).
Later on, I use the Strategy Pattern to define different movement and shooting controllers which implement different behaviours.
Comments